In your applications, you may want to pass custom query string parameters to an entity form.
Let me describe the way in which we can define a set of specific parameter names and data types that can be accepted for a specific entity form.
Define Allowed Query String Parameters:There are two ways to specify which query string parameters will be accepted by the form:
• Edit form properties
• Edit form XML
Edit Form Properties:When you edit an entity form, on the Home tab in the Form group, click Form Properties. In the Form Properties dialog box, select the Parameters tab. Use this tab to modify the names and data types that the form allows as given in the below screen shot.
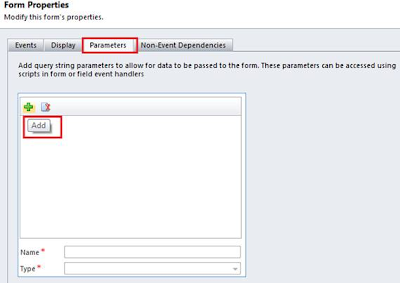
The following describes the querystringparameter element attributes, name and type:
Name: Each name attribute must contain at least one underscore ('_') character, but the name of the query string parameter cannot begin with an underscore. The name also cannot start with 'crm_'. We strongly recommend that you use the customization prefix of the solution publisher as the naming convention. If you don’t follow the naming conventions the alert message as given in the below screen shot will be shown to the user.
Type: Following types of parameters can be passed to the form. Match the data type values with the parameter values so that invalid data is not passed with the parameter. The following are valid data types:
• Boolean
• DateTime
• Double
• EntityType
• Integer
• Long
• PositiveInteger
• SafeString
• UniqueId
• UnsignedInt
Let me define the parameters for the form and access them through Query string parameters.
- We have created two parameters for the Account Form as given in the below screen shot.
- We have opened the form through the below given script with parameters appended to the URL.
var extraqs = "Parameter_Source=Hello";
extraqs += "parameter_Source2=8";
//Set features for how the window will appear.
var features = "location=no,menubar=no,status=no,toolbar=no";
// Open the window.
window.open(Xrm.Page.context.getServerUrl() +"/main.aspx?etn=account&pagetype=entityrecord&extraqs=" + encodeURIComponent(extraqs), "_blank", features, false);
- After that we have checked it through the query string parameters.
// Get the Query String Parametrs passedthrough the URL
var param=context.getQueryStringParameters();
// Get the Value of the Source through the Key Parameter Name
var source=param["Parameter_Source"];
var source2=param["parameter_Source2"];
// if Source is Undefined i.e. the URL Doesn't contain the key
if (source==undefined){ return; }
else{ // Perform the operation
}
This will be helpful in the scenario suppose if are opening the Form through the different sources such as Silverlight, report etc. and on the form load you want to perform some operation based on the source then you can define parameter for the form and can pass the source name in the extraqs of the URL as mentioned above and can check the source name on the form load and can perform the operation accordingly.